给定一个字符串,请你找出其中不含有重复字符的 *最长子串 *的长度。
示例 1:
输入: “abcabcbb”
输出: 3
解释: 因为无重复字符的最长子串是 "abc",所以其
长度为 3。
示例 2:
输入: “bbbbb”
输出: 1
解释: 因为无重复字符的最长子串是 "b"
,所以其长度为 1。
示例 3:
输入: “pwwkew”
输出: 3
解释: 因为无重复字符的最长子串是 "wke"
,所以其长度为 3。
请注意,你的答案必须是 子串 的长度,"pwke"
是一个子序列,不是子串。
利用滑动窗口求解
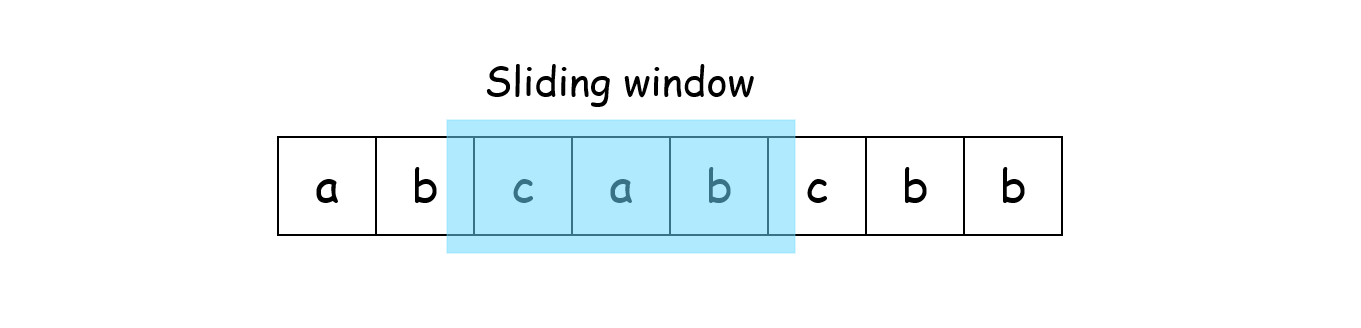
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| class Solution { public int lengthOfLongestSubstring(String s) { int head = 0; int max = 0;
char[] chars = s.toCharArray(); HashSet<Character> stringHashSet = new HashSet<>(); for (int tail = 0; tail < chars.length; tail++) { if (!stringHashSet.contains(chars[tail])) { max = Math.max(max, tail - head +1); }else { while (stringHashSet.contains(chars[tail])) { stringHashSet.remove(chars[head]); head++; } } stringHashSet.add(chars[tail]); } return max; }
}
|